版本:Python3.6
系統:Windows
相關模組:
-
Requests
-
BeautifulSoup4
-
Selenium (配置好Chrome Driver、Firefox Driver或是PhantomJS環境)
京東賬號得關聯QQ,且當前QQ線上 (用於QQ授權登入京東,可自行擴充套件登入方式)
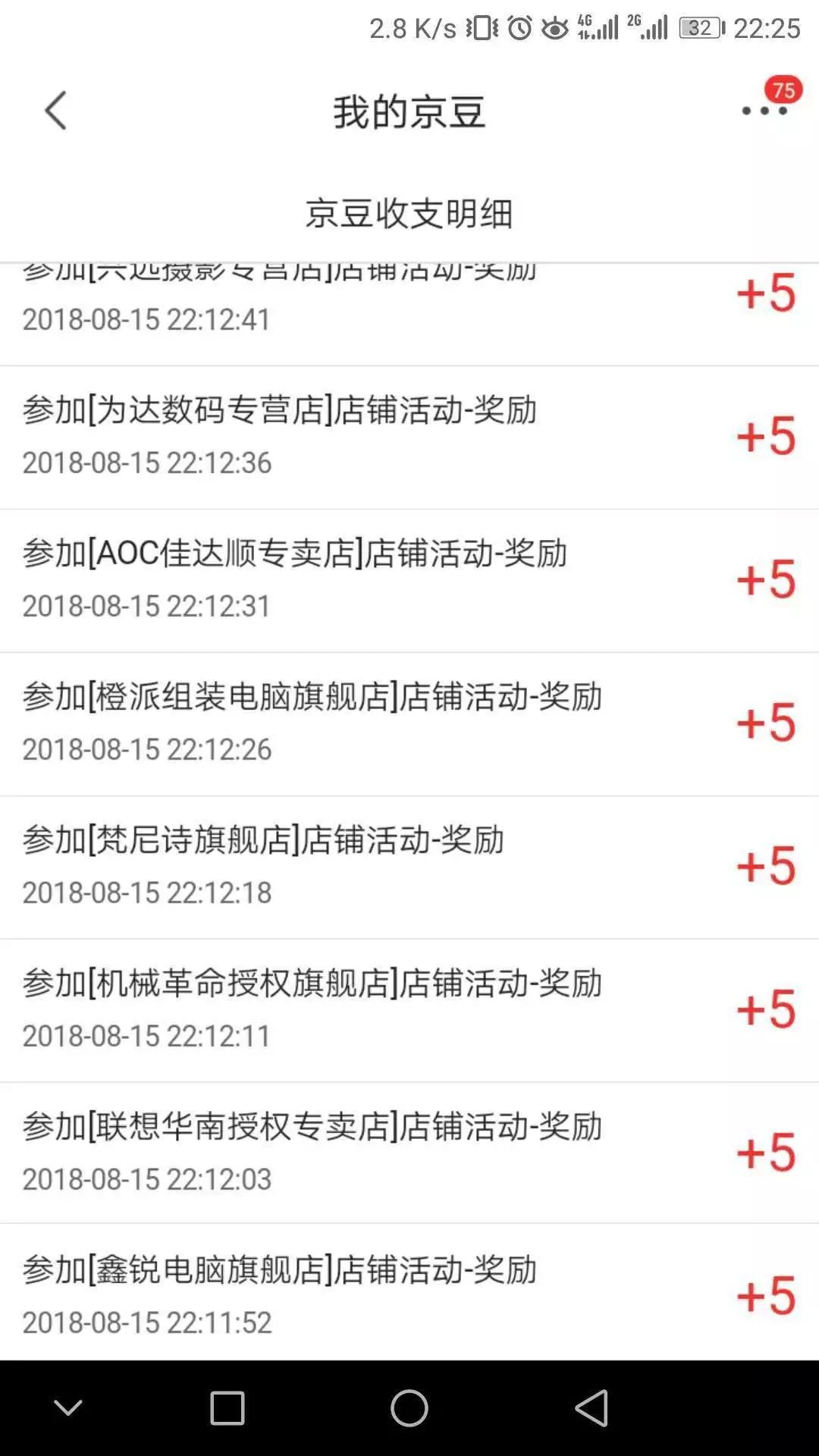
wx_turing.py
import time
from urllib.parse import parse_qsimport requests
from bs4 import BeautifulSoup
from selenium import webdriver
from selenium.common.exceptions import *
from selenium.webdriver.support.wait import WebDriverWait# 額外抽取的授權模組
from utils import authclass QMM(object):
“””藉助券媽媽平臺褥京東京豆”””def __init__(self, sleep=3, months=None, days=None):
self.timeout, self.months, self.days = sleep, None, None
# 爬取規則
if months:
month_interval = months.split(‘-‘)
start_month, end_month = int(month_interval[0]), int(month_interval[-1])
self.months = list(map(lambda m: ‘{}月’.format(m), range(start_month, end_month + 1)))
if days:
day_interval = days.split(‘-‘)
start_day, end_day = int(day_interval[0]), int(day_interval[-1])
self.days = list(map(lambda d: ‘{}日’.format(d), range(start_day, end_day + 1)))
# 手機店鋪(用作提醒輸出,可複製連結到手機端領取)
self.m_shop = []
# 統計京豆總數
self.jing_dou = 0def _crawl_url(self):
“”” 抓取京豆更新頁, 獲得店鋪京豆領取地址”””# 日期更新頁
qmm_collect = ‘http://www.quanmama.com/zhidemai/2459063.html’
bs = BeautifulSoup(requests.get(qmm_collect).text, ‘html.parser’)
for link in bs.tbody.find_all(‘a’):
text = link.text
if self.months:
if not list(filter(lambda m: m in text, self.months)): continue
if self.days:
if not list(filter(lambda d: d in text, self.days)): continueqmm_detail = link.get(‘href’)
# 店鋪領取頁
resp = requests.get(qmm_detail)
bs = BeautifulSoup(resp.text, ‘html.parser’)
for body in bs.find_all(‘tbody’):
for mall in body.find_all(‘a’):
url = self._parse_url(mall.get(‘href’))
if ‘shop.m.jd.com’ in url:
self.m_shop.append(url)
else:
yield url@staticmethod
def _parse_url(url):
“””提取URL中的url引數”””mall_url = parse_qs(url).get(‘url’)
return mall_url.pop() if mall_url else urldef start(self):
“”” 登入京東,領取店鋪羊毛”””malls = set(self._crawl_url())
print(‘共有 %d 個可褥羊毛PC端店鋪頁面’ % len(malls))m_malls = self.m_shop
print(‘共有 %d 個可褥羊毛手機端店鋪頁面’ % len(m_malls))
for m_mall in m_malls:
print(m_mall)if malls:
# 登陸京東(Chrome、PhantomJS or FireFox)
driver = webdriver.Chrome() # driver = webdriver.PhantomJS()
jd_login = ‘https://passport.jd.com/new/login.aspx’
driver.get(jd_login)# 視窗最大化
driver.maximize_window()# QQ授權登入
driver.find_element_by_xpath(‘//*[@id=”kbCoagent”]/ul/li[1]/a’).click()
auth.qq(driver)
time.sleep(self.timeout)# 開始褥羊毛
for i, detail in enumerate(malls):
driver.get(detail)
print(‘%d.店鋪: %s’ % (i + 1, detail), end=”)
try:
# 查詢”領取”按鈕
btn = WebDriverWait(driver, self.timeout).until(
lambda d: d.find_element_by_css_selector(“[class=’J_drawGift d-btn’]”))
except TimeoutException:
# 失敗大多數情況下是無羊毛可褥(券媽媽平臺只是簡單彙總但不一定就有羊毛)
print(‘ 領取失敗, TimeoutException ‘)
else:
try:
# 輸出羊毛戰績
items = WebDriverWait(driver, self.timeout).until(
lambda d: d.find_elements_by_css_selector(“[class=’d-item’]”))
for item in items:
item_type = item.find_element_by_css_selector(“[class=’d-type’]”).text
item_num = item.find_element_by_css_selector(“[class=’d-num’]”).text
if item_type == ‘京豆’: self.jing_dou += item_num
print(‘ {}{} ‘.format(item_type, item_num), end=”)
except:
# 此處異常不太重要, 忽略
pass
finally:
btn.click()
print(‘ 領取成功’)# 以下附加功能可選
self._print_jing_dou()
self._un_subscribe(driver)
self._finance_sign(driver)def _print_jing_dou(self):
print(‘O(∩_∩)O哈哈~, 共褥到了{}個京豆,相當於RMB{}元’, self.jing_dou, self.jing_dou / 100)def _un_subscribe(self, driver):
“””批次取消店鋪關註”””# 進入關註店鋪
subscribe_shop = ‘https://t.jd.com/vender/followVenderList.action’
driver.get(subscribe_shop)try:
# 批次操作
batch_btn = WebDriverWait(driver, self.timeout).until(
lambda d: d.find_element_by_xpath(‘//*[@id=”main”]/div/div[2]/div[1]/div[2]/div[2]/div/a’))
batch_btn.click()
# 全選店鋪
all_btn = WebDriverWait(driver, self.timeout).until(
lambda d: d.find_element_by_xpath(‘//*[@id=”main”]/div/div[2]/div[1]/div[2]/div[2]/div/div/span[1]’))
all_btn.click()
# 取消關註
cancel_btn = WebDriverWait(driver, self.timeout).until(
lambda d: d.find_element_by_xpath(‘//*[@id=”main”]/div/div[2]/div[1]/div[2]/div[2]/div/div/span[2]’))
cancel_btn.click()
# 彈框確認
confirm_btn = WebDriverWait(driver, self.timeout).until(
lambda d: d.find_element_by_xpath(“/html/body/div[7]/div[3]/a[1]”))
except TimeoutException:
print(‘ 批次取關店鋪失敗, TimeoutException ‘)
else:
confirm_btn.click()
print(‘ 已批次取消關註店鋪’)def _finance_sign(self, driver):
“””京東金融簽到領鋼鏰”””# 進入京東金融
jr_login = ‘https://jr.jd.com/’
driver.get(jr_login)try:
# 點選簽到按鈕
sign_btn = WebDriverWait(driver, self.timeout).until(
lambda d: d.find_element_by_xpath(‘//*[@id=”primeWrap”]/div[1]/div[3]/div[1]/a’))
except TimeoutException:
print(‘ 京東金融簽到失敗, TimeoutException ‘)
else:
sign_btn.click()
print(‘ 京東金融簽到成功’)if __name__ == ‘__main__’:
qmm = QMM(sleep=3, months=‘7-8’, days=’16-31′)
qmm.start()
(左右滑動即可檢視完整程式碼)
auth.py
from selenium.webdriver.support.wait import WebDriverWait
# QQ授權登入, 使用前提是QQ客戶端線上
def qq(driver, timeout=3):
# 切換到最新開啟的視窗
window_handles = driver.window_handles
driver.switch_to.window(window_handles[-1])print(‘Auth QQ: ‘, driver.title)
# 切換iframe
i_frame = WebDriverWait(driver, timeout).until(lambda d: d.find_element_by_id(‘ptlogin_iframe’))
driver.switch_to.frame(i_frame)# 點選頭像進行授權登入
login = WebDriverWait(driver, timeout).until(lambda d: d.find_element_by_xpath(‘//*[@id=”qlogin_list”]/a[1]’))
login.click()
(左右滑動即可檢視完整程式碼)
該功能還能繼續完善一下的:
- 加入每日定時功能
- 擴充套件登入京東方式
- 多執行緒褥羊毛(需求不大)
- Appium抓取手機店鋪主頁
對於這次拼多多薅羊毛漏洞問題,各位看官,你覺得拼多多該全責埋單,還是給予補償來修複已經被擴大到200億級的漏洞呢?
不妨留個言討論討論。