
作者 | Likegeeks
譯者 | ghsgz
在本文中,我們將看一些 TensorFlow 的例子,並從中感受到在定義張量和使用張量做數學計算方面有多麼容易,我還會舉些別的機器學習相關的例子。
TensorFlow 是什麼?
TensorFlow 是 Google 為瞭解決複雜的數學計算耗時過久的問題而開發的一個庫。
事實上,TensorFlow 能幹許多事。比如:
開始寫例子前,需要瞭解一些基本知識。
什麼是張量?
張量是 TensorFlow 使用的主要的資料塊,它類似於變數,TensorFlow 使用它來處理資料。張量擁有維度和型別的屬性。
維度指張量的行和列數,讀到後面你就知道了,我們可以定義一維張量、二維張量和三維張量。
型別指張量元素的資料型別。
定義一維張量
可以這樣來定義一個張量:建立一個 NumPy 陣列(LCTT 譯註:NumPy 系統是 Python 的一種開源數字擴充套件,包含一個強大的 N 維陣列物件 Array,用來儲存和處理大型矩陣 )或者一個 Python 串列[1] ,然後使用 tf_convert_to_tensor
函式將其轉化成張量。
可以像下麵這樣,使用 NumPy 建立一個陣列:
import numpy as np arr = np.array([1, 5.5, 3, 15, 20])
arr = np.array([1, 5.5, 3, 15, 20])
執行結果顯示了這個陣列的維度和形狀。
import numpy as np
arr = np.array([1, 5.5, 3, 15, 20])
print(arr)
print(arr.ndim)
print(arr.shape)
print(arr.dtype)
它和 Python 串列很像,但是在這裡,元素之間沒有逗號。
現在使用 tf_convert_to_tensor
函式把這個陣列轉化為張量。
import numpy as np
import tensorflow as tf
arr = np.array([1, 5.5, 3, 15, 20])
tensor = tf.convert_to_tensor(arr,tf.float64)
print(tensor)
這次的執行結果顯示了張量具體的含義,但是不會展示出張量元素。
要想看到張量元素,需要像下麵這樣,執行一個會話:
import numpy as np
import tensorflow as tf
arr = np.array([1, 5.5, 3, 15, 20])
tensor = tf.convert_to_tensor(arr,tf.float64)
sess = tf.Session()
print(sess.run(tensor))
print(sess.run(tensor[1]))
定義二維張量
定義二維張量,其方法和定義一維張量是一樣的,但要這樣來定義陣列:
arr = np.array([(1, 5.5, 3, 15, 20),(10, 20, 30, 40, 50), (60, 70, 80, 90, 100)])
接著轉化為張量:
import numpy as np
import tensorflow as tf
arr = np.array([(1, 5.5, 3, 15, 20),(10, 20, 30, 40, 50), (60, 70, 80, 90, 100)])
tensor = tf.convert_to_tensor(arr)
sess = tf.Session()
print(sess.run(tensor))
現在你應該知道怎麼定義張量了,那麼,怎麼在張量之間跑數學運算呢?
在張量上進行數學運算
假設我們有以下兩個陣列:
arr1 = np.array([(1,2,3),(4,5,6)])
arr2 = np.array([(7,8,9),(10,11,12)])
利用 TenserFlow ,你能做許多數學運算。現在我們需要對這兩個陣列求和。
使用加法函式來求和:
import numpy as np
import tensorflow as tf
arr1 = np.array([(1,2,3),(4,5,6)])
arr2 = np.array([(7,8,9),(10,11,12)])
arr3 = tf.add(arr1,arr2)
sess = tf.Session()
tensor = sess.run(arr3)
print(tensor)
也可以把陣列相乘:
import numpy as np
import tensorflow as tf
arr1 = np.array([(1,2,3),(4,5,6)])
arr2 = np.array([(7,8,9),(10,11,12)])
arr3 = tf.multiply(arr1,arr2)
sess = tf.Session()
tensor = sess.run(arr3)
print(tensor)
現在你知道了吧。
三維張量
我們已經知道了怎麼使用一維張量和二維張量,現在,來看一下三維張量吧,不過這次我們不用數字了,而是用一張 RGB 圖片。在這張圖片上,每一塊畫素都由 x、y、z 組合表示。
這些組合形成了圖片的寬度、高度以及顏色深度。
首先使用 matplotlib 庫匯入一張圖片。如果你的系統中沒有 matplotlib ,可以 使用 pip[2]來安裝它。
將圖片放在 Python 檔案的同一目錄下,接著使用 matplotlib 匯入圖片:
import matplotlib.image as img
myfile = "likegeeks.png"
myimage = img.imread(myfile)
print(myimage.ndim)
print(myimage.shape)
從執行結果中,你應該能看到,這張三維圖片的寬為 150 、高為 150 、顏色深度為 3 。
你還可以檢視這張圖片:
import matplotlib.image as img
import matplotlib.pyplot as plot
myfile = "likegeeks.png"
myimage = img.imread(myfile)
plot.imshow(myimage)
plot.show()
真酷!
那怎麼使用 TensorFlow 處理圖片呢?超級容易。
使用 TensorFlow 生成或裁剪圖片
首先,向一個佔位符賦值:
myimage = tf.placeholder("int32",[None,None,3])
使用裁剪操作來裁剪影象:
cropped = tf.slice(myimage,[10,0,0],[16,-1,-1])
最後,執行這個會話:
result = sess.run(cropped, feed\_dict={slice: myimage})
然後,你就能看到使用 matplotlib 處理過的影象了。
這是整段程式碼:
import tensorflow as tf
import matplotlib.image as img
import matplotlib.pyplot as plot
myfile = "likegeeks.png"
myimage = img.imread(myfile)
slice = tf.placeholder("int32",[None,None,3])
cropped = tf.slice(myimage,[10,0,0],[16,-1,-1])
sess = tf.Session()
result = sess.run(cropped, feed_dict={slice: myimage})
plot.imshow(result)
plot.show()
是不是很神奇?
使用 TensorFlow 改變影象
在本例中,我們會使用 TensorFlow 做一下簡單的轉換。
首先,指定待處理的影象,並初始化 TensorFlow 變數值:
myfile = "likegeeks.png"
myimage = img.imread(myfile)
image = tf.Variable(myimage,name='image')
vars = tf.global_variables_initializer()
然後呼叫 transpose 函式轉換,這個函式用來翻轉輸入網格的 0 軸和 1 軸。
sess = tf.Session()
flipped = tf.transpose(image, perm=[1,0,2])
sess.run(vars)
result=sess.run(flipped)
接著你就能看到使用 matplotlib 處理過的影象了。
import tensorflow as tf
import matplotlib.image as img
import matplotlib.pyplot as plot
myfile = "likegeeks.png"
myimage = img.imread(myfile)
image = tf.Variable(myimage,name='image')
vars = tf.global_variables_initializer()
sess = tf.Session()
flipped = tf.transpose(image, perm=[1,0,2])
sess.run(vars)
result=sess.run(flipped)
plot.imshow(result)
plot.show()
以上例子都向你表明瞭使用 TensorFlow 有多麼容易。
via: https://www.codementor.io/likegeeks/define-and-use-tensors-using-simple-tensorflow-examples-ggdgwoy4u
作者:LikeGeeks[4] 譯者:ghsgz 校對:wxy
本文由 LCTT 原創編譯,Linux中國 榮譽推出
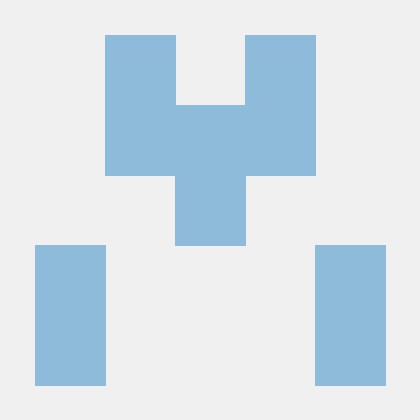