作者:丶E
連結:https://www.jianshu.com/p/48a89e678d6c
-
先看小紅書的效果
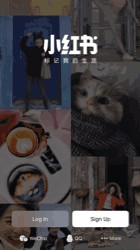
-
說說思路
滾動效果用RecyclerView實現。RecyclerView有個smoothScrollToPosition方法,可以滾動到指定位置(有滾動效果,不是直接到指定位置),不瞭解的看這裡RecycleView4種定位滾動方式演示。每一個Item是一張長圖,這樣首尾相接滾動起來(滾到無限遠)就是無限迴圈的效果,然後再改變滾動的速度,完成。
public class MainActivity extends AppCompatActivity {
private RecyclerView mRecyclerView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
//全屏
getWindow().setFlags(WindowManager.LayoutParams.FLAG_FULLSCREEN, WindowManager.LayoutParams.FLAG_FULLSCREEN);
setContentView(R.layout.activity_main);
mRecyclerView = findViewById(R.id.mRecyclerView);
mRecyclerView.setAdapter(new SplashAdapter(MainActivity.this));
mRecyclerView.setLayoutManager(new ScollLinearLayoutManager(MainActivity.this));
//smoothScrollToPosition滾動到某個位置(有滾動效果)
mRecyclerView.smoothScrollToPosition(Integer.MAX_VALUE / 2);
}
}
-
無限迴圈
將RecyclerView的Item數量設定成很大的值,用smoothScrollToPosition方法滾到很遠的位置,就能實現這樣的效果,很多banner輪播圖的實現也是如此;
public class SplashAdapter extends RecyclerView.Adapter<SplashAdapter.ViewHolder> {
private int imgWidth;
public SplashAdapter(Context context) {
imgWidth = EasyUtil.getScreenWidth(context);
}
@Override
public ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View itemView = LayoutInflater.from(parent.getContext()).inflate(R.layout.item_splash, parent, false);
return new ViewHolder(itemView);
}
@Override
public void onBindViewHolder(final ViewHolder holder, final int position) {
/* ViewGroup.LayoutParams lp = holder.item_bg.getLayoutParams();
lp.width = imgWidth;
lp.height =imgWidth*5;
holder.item_bg.setLayoutParams(lp);*/
}
@Override
public int getItemCount() {
return Integer.MAX_VALUE;
}
public class ViewHolder extends RecyclerView.ViewHolder {
ImageView item_bg;
public ViewHolder(final View itemView) {
super(itemView);
item_bg = itemView.findViewById(R.id.item_bg);
}
}
}
-
控制smoothScrollToPosition的滑動速度
參考RecyclerView呼叫smoothScrollToPosition() 控制滑動速度,修改MILLISECONDS_PER_INCH的值即可
/**
* 更改RecyclerView滾動的速度
*/
public class ScollLinearLayoutManager extends LinearLayoutManager {
private float MILLISECONDS_PER_INCH = 25f; //修改可以改變資料,越大速度越慢
private Context contxt;
public ScollLinearLayoutManager(Context context) {
super(context);
this.contxt = context;
}
@Override
public void smoothScrollToPosition(RecyclerView recyclerView, RecyclerView.State state, int position) {
LinearSmoothScroller linearSmoothScroller =
new LinearSmoothScroller(recyclerView.getContext()) {
@Override
public PointF computeScrollVectorForPosition(int targetPosition) {
return ScollLinearLayoutManager.this
.computeScrollVectorForPosition(targetPosition);
}
@Override
protected float calculateSpeedPerPixel
(DisplayMetrics displayMetrics) {
return MILLISECONDS_PER_INCH / displayMetrics.density;
//傳回滑動一個pixel需要多少毫秒
}
};
linearSmoothScroller.setTargetPosition(position);
startSmoothScroll(linearSmoothScroller);
}
//可以用來設定速度
public void setSpeedSlow(float x) {
//自己在這裡用density去乘,希望不同解析度裝置上滑動速度相同
//0.3f是自己估摸的一個值,可以根據不同需求自己修改
MILLISECONDS_PER_INCH = contxt.getResources().getDisplayMetrics().density * 0.3f + (x);
}
}
-
圖片寬度充滿螢幕、高度按圖片原始寬高比例自適應
@SuppressLint("AppCompatCustomView")
public class FitImageView extends ImageView {
public FitImageView(Context context) {
super(context);
}
public FitImageView(Context context, AttributeSet attrs) {
super(context, attrs);
}
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec){
Drawable drawable = getDrawable();
if(drawable!=null){
int width = MeasureSpec.getSize(widthMeasureSpec);
int height = (int) Math.ceil((float) width * (float) drawable.getIntrinsicHeight() / (float) drawable.getIntrinsicWidth());
setMeasuredDimension(width, height);
}else{
super.onMeasure(widthMeasureSpec, heightMeasureSpec);
}
}
}
-
這裡需要註意的是、Item的根佈局android:layout_height=”wrap_content”,否則圖片高度會受限
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content">
<com.next.scrollimagedemo.view.FitImageView
android:id="@+id/item_bg"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:src="@mipmap/ww1" />
android.support.constraint.ConstraintLayout>
-
使RecyclerView不能手指觸碰滑動
加層View遮蔽掉事件就好了
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity">
<android.support.v7.widget.RecyclerView
android:id="@+id/mRecyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent">android.support.v7.widget.RecyclerView>
<TextView
android:layout_width=“match_parent”
android:layout_height=“match_parent”
android:background=“#80000000”
android:clickable=“true” />
<ImageView
android:layout_width=“wrap_content”
android:layout_height=“80dp”
android:layout_marginTop=“80dp”
app:layout_constraintTop_toTopOf=“parent”
android:scaleType=“centerInside”
android:src=“@mipmap/slogan”
app:layout_constraintLeft_toLeftOf=“parent”
app:layout_constraintRight_toRightOf=“parent” />
android.support.constraint.ConstraintLayout>
-
完成效果

-
Demo
github:https://github.com/forvv231/EasyScollImage
apk:https://fir.im/gfdj
-
By the way
不要吐槽這背景圖片、不喜歡、Demo程式碼裡面還有別的( ̄▽ ̄)~*
●編號367,輸入編號直達本文
●輸入m獲取到文章目錄
Java程式設計
更多推薦《18個技術類公眾微信》
涵蓋:程式人生、演演算法與資料結構、駭客技術與網路安全、大資料技術、前端開發、Java、Python、Web開發、安卓開發、iOS開發、C/C++、.NET、Linux、資料庫、運維等。